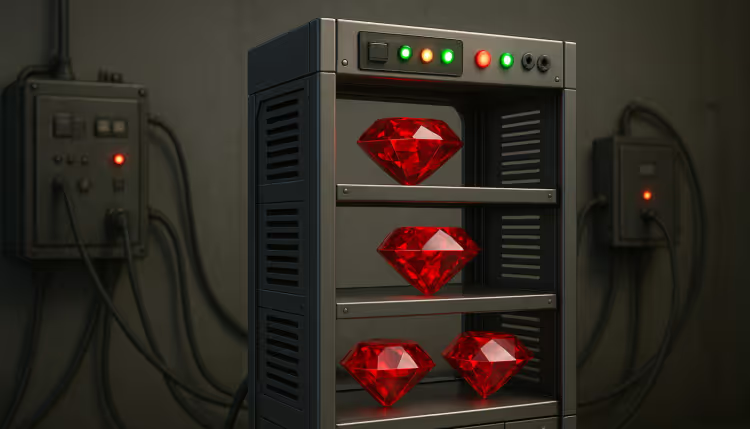
Ruby Libraries
One of Ruby’s greatest strengths lies in its extensive library ecosystem, which provides ready-to-use tools for various tasks. These libraries fall into three main categories: Core Library, Standard Library, and RubyGems.
1. Ruby Core Library
The Core Library includes fundamental features that are built into Ruby. You don’t need to install or require them—they are always accessible.
Some examples:
- Strings and Arrays:
upcase
,sum
,reverse
- Enumerables:
map
,select
,reduce
Example using upcase
for strings
name = "Ruby"puts name.upcase # Outputs "RUBY"
2. Ruby Standard Library
The Standard Library is also included with Ruby, and it extends the language with additional functionality. The main difference is that you need to explicitly require these tools before using them.
Some examples:
date
– Work with datesdigest
– Encrypt datajson
– Handle JSON datanet/http
– Make web requests
Example using json
to handle data
require 'json'
data = { name: 'Alice', age: 25 }JSON.generate(data) # Convert hash to JSON
Note that this library is automatically loaded in the Ruby console (irb
), so you don’t need to do the require
.
3. RubyGems
RubyGems is a repository that provides thousands of additional libraries (gems) for specialized tasks. These gems must be installed and required before use.
Some examples:
nokogiri
– HTML/XML parsingsinatra
– Lightweight web frameworkrails
– Full-featured web development framework
Example using sinatra
to build a minimal web app
First install the gem:
gem install sintra
Then you can require and use it:
require 'sinatra'
get '/' do 'Hello world!'end
Summary
Ruby offers three types of libraries, each serving a distinct purpose:
- Core Library: Provides built-in, essential features for handling data structures and fundamental operations.
- Standard Library: Expands functionality with additional modules for tasks like date manipulation, encryption, and network communication.
- RubyGems: A vast collection of external libraries (gems), usually for more complex needs, like a complete authentication system for a website.