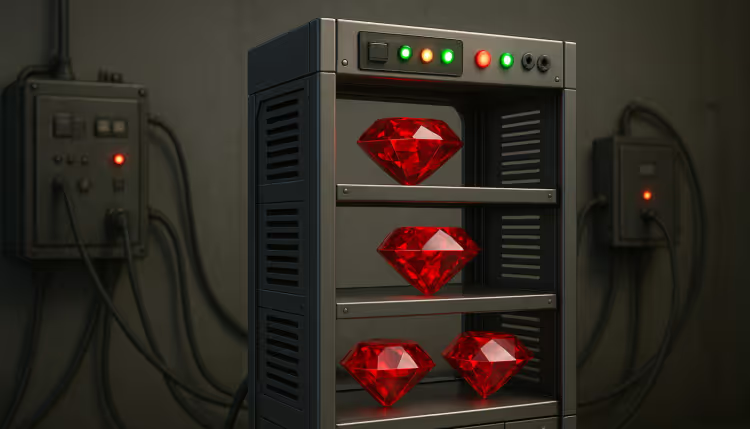
What is Ruby?
Curious why many developers use Ruby? You may have heard about its elegant syntax, dynamic features, and the supportive community behind it. Ruby is often described as intuitive to read and write, allowing you to focus on problem-solving rather than dealing with overly strict rules.
Developed in the mid-1990s by Yukihiro “Matz” Matsumoto, Ruby was designed to make programming more enjoyable while remaining practical. Matz expressed his vision by saying, “I want to make Ruby natural, not simple.” This perspective guided the language’s growth, aiming for a balance between clarity and versatility. Instead of stripping away features for simplicity, Ruby encourages expressiveness and flexibility, making it suitable for a range of tasks.
Everything is an Object
One of Ruby’s core principles is that everything is treated as an object. Integers, strings, and even classes themselves are all objects with their own methods and properties. This unified approach can feel more consistent compared to languages that treat certain data types as “primitive” or separate. For example:
# Integers are objectsputs 5.class # => Integerputs 5.next # => 6
# Strings are objectsputs "Ruby".class # => Stringputs "Ruby".reverse # => "ybuR"
# Classes are also objectsputs String.class # => Class
Because everything in Ruby is an object, you have a consistent, object-oriented way of coding across the board. You can even extend built-in classes directly, which highlights how flexible the language can be:
class Integer def triple self * 3 endend
puts 4.triple # => 12
Here, Integer
is just another Ruby object that you can open up and modify.
Flexibility
In Ruby, operators like +
and -
are not special cases baked into the language, they are just methods. For instance, let’s compare the shorthand +
operator with its explicit method call:
# Summing two numbers with the '+' operator2 + 3 # => 5
# Summing two numbers by calling the '+' method2.+(3) # => 5
In Ruby, both approaches accomplish the same thing, because the +
operator is just a method call under the hood. This design is what makes it possible to redefine operators for custom classes. For example:
class Greeting attr_accessor :message
def initialize(message) @message = message end
# Redefine '+' to concatenate two Greetings def +(other) Greeting.new("#{@message} #{other.message}") endend
hello = Greeting.new("Hello")world = Greeting.new("World!")combined = hello + world
puts combined.message # => "Hello World!"
When you use the +
operator, Ruby calls the +
method, letting you decide how two Greeting
objects should combine. Here, we simply create a new Greeting
whose message is the concatenation of the two originals.
Blocks: Ruby’s Secret Sauce
A block in Ruby is a chunk of code enclosed between do...end
or curly braces {...}
. You can pass these blocks to methods, which can then decide how to execute the code inside. For example:
[1, 2, 3].each do |number| puts numberend
Here, the block do |number| ... end
is passed to the each
method, which will run the block for each element in the array.
Blocks often allow for shorter, more expressive code because you define what you want to do in one place, without adding extra method definitions:
# Print "Hello, Ruby!" three times3.times { puts "Hello, Ruby!" }
In this snippet, the block { puts "Hello, Ruby!" }
defines what happens for each iteration.
Sharing Behavior with Mixins
Ruby doesn’t allow multiple inheritance, but you can still share methods across multiple classes by using mixins. Simply place your common code in a module, then include that module in any class that needs it:
module Flyable def fly "I'm flying!" endend
class Bird include Flyableend
puts Bird.new.fly # => "I'm flying!"
Mixins keep your classes simpler by letting you group related methods in one place and “mix” them into any class, avoiding a complicated inheritance hierarchy.
Clarity
One of Ruby’s design goals is to emphasize clarity throughout the code. For instance, Ruby uses question marks (?
) in method names that return a boolean result, and exclamation marks (!
) for methods that modify an object in place:
str = "Ruby"
puts str.empty? # => falseputs str.upcase! # => "RUBY"
Another example that brings clarity and expressiveness is the place where you can place the conditionals. For instance, you can place an if
condition after a statement instead of wrapping it in a block:
messages = ["Hello", "Hi!"]puts "You have new messages!" if messages.any?
This postfix conditional reads much like a natural sentence, helping you see the core idea at a glance.
Under the Hood
Ruby manages memory through a mark-and-sweep garbage collector. When you create objects, they remain in memory as long as they’re referenced. Once they’re no longer needed, Ruby’s garbage collector identifies and frees them automatically. This system helps you focus on writing your code rather than dealing with manual memory management.
Another key feature is Ruby’s ability to run on various operating systems, such as Linux, macOS, and Windows. This flexibility means you can develop on your preferred environment without worrying about compatibility across different machines or servers.
Where Ruby Shines
Web Development
Ruby is closely associated with web development, largely thanks to the Ruby on Rails framework. Rails offers a “convention over configuration” philosophy, which can reduce repetitive setup work in building websites and services. While other frameworks exist, Rails remains a popular choice for quickly creating database-driven applications.
Scripting & Automation
Ruby’s straightforward syntax and numerous built-in methods make it handy for writing quick scripts. Whether you’re automating file tasks, parsing data, or gluing different services together, Ruby offers a clean, readable way to get the job done without a lot of setup.
Prototype-Friendly
Ruby’s blocks, metaprogramming features, and flexible syntax also cater to rapid prototyping. You can add or modify methods at runtime, create domain-specific languages (DSLs), or leverage blocks for custom iteration and callbacks—all of which can simplify experimentation and speed up development.
Getting Started
If you’re new to Ruby, the first step is installing it on your machine. Once installed, try experimenting with the Interactive Ruby Shell (IRB). Simply open your terminal and type:
irb
From there, you can type Ruby expressions, run them immediately, and get a feel for the language in a hands-on way.
Community & Resources
Ruby’s community is known for being approachable and resourceful. You can join mailing lists, participate in online forums or chats, listen to podcasts, or attend local and international Ruby conferences to meet others who share your interests.
These gatherings and discussion channels are great places to ask questions, learn best practices, and stay updated on the latest developments in Ruby.
Conclusion
Ruby’s design blends a friendly, human-centered approach with practical features that help you solve problems efficiently. It offers a clear syntax, flexible tools like blocks and mixins, and a supportive community.
If you’re curious, the best next step is to install Ruby, try a few lines of code in IRB, and explore the official documentation. By experimenting with real examples, you can discover how Ruby’s thoughtful design can make programming both productive and enjoyable.