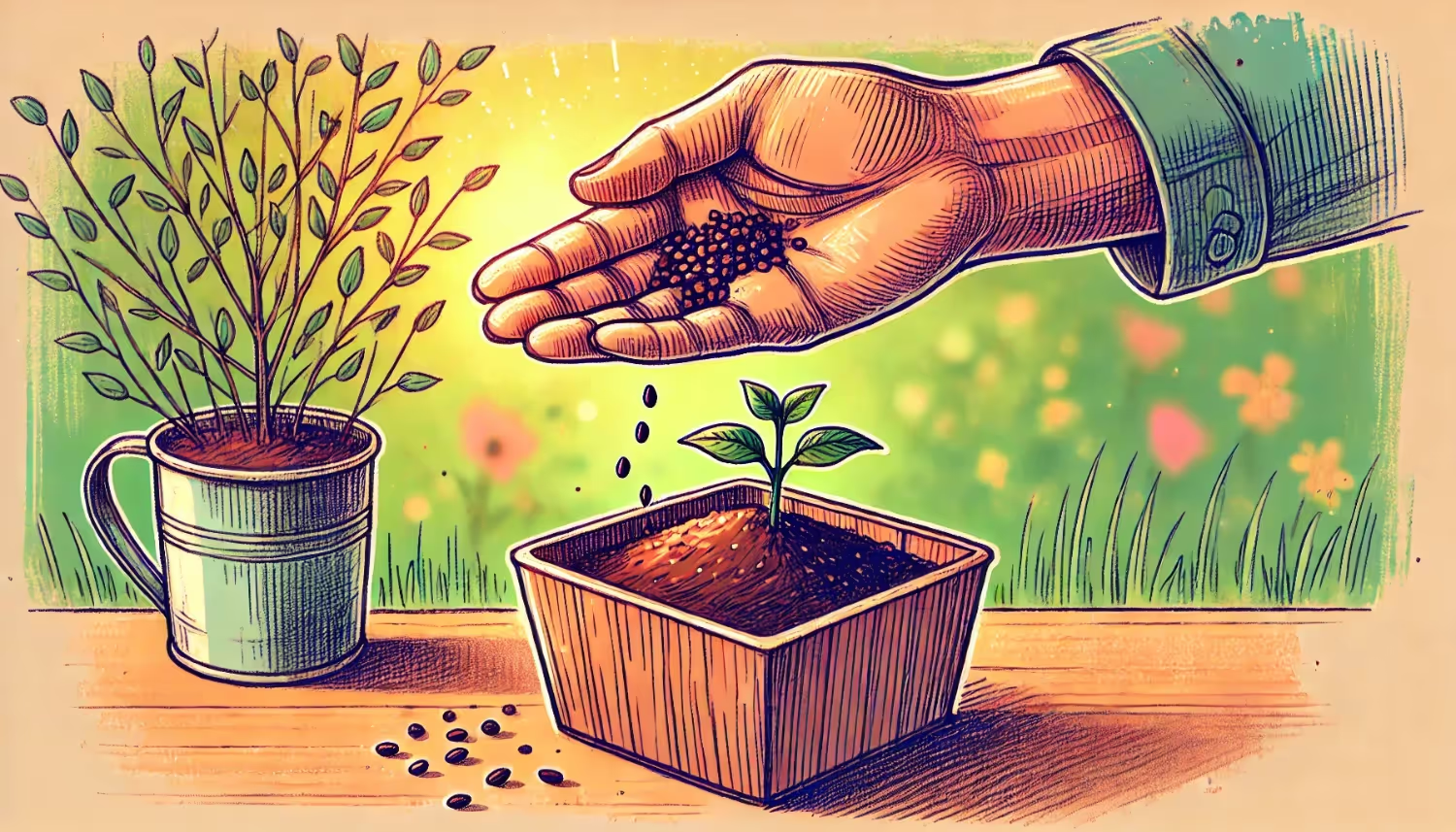
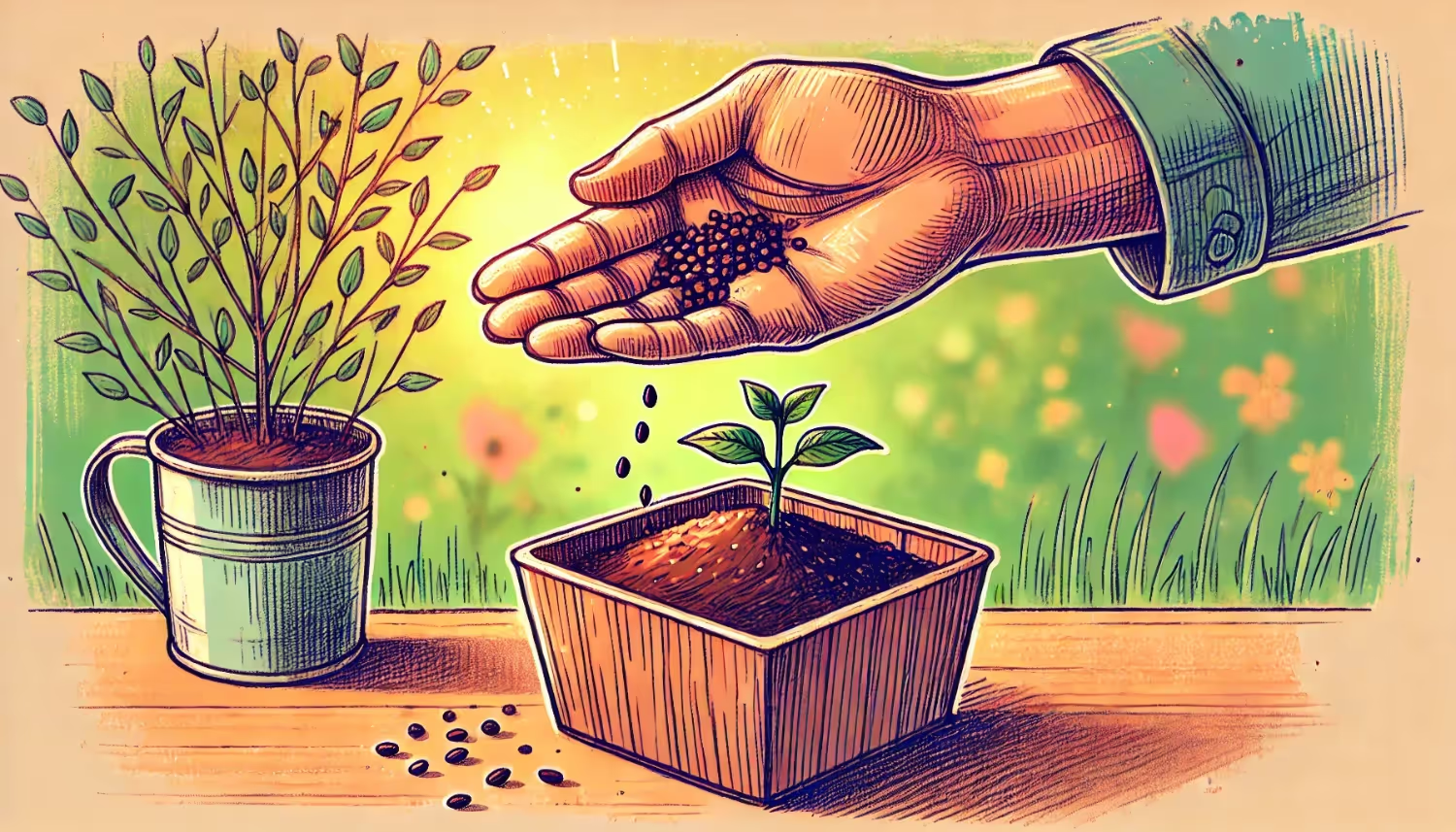
Understanding Active Record
Active Record is an architectural pattern that bridges the gap between in-memory object data and relational databases such as MySQL or PostgreSQL. This way, database interactions can be handled without having to write direct SQL queries.
What Is Active Record?
Active Record is the backbone of the model layer in the Model-View-Controller (MVC) architecture, which is responsible for managing data and business logic. In frameworks like Ruby on Rails, Active Record is used for building Ruby objects that require persistent storage in a relational database.
Essentially, each Active Record object corresponds to a specific row in a database table. It also contains methods to interact with it, such as creating, reading, updating, and deleting records.
This simplicity allows to easily read and write data while maintaining consistency between application objects and their corresponding database record.
Active Record vs. Active Model
Active Record is often confused with Active Model, which is also part of the model layer in Rails. Here is a brief summary of their differences:
-
Active Record: deals with objects backed by a database, handling persistence and database operations.
-
Active Model: provides functionality for modeling data without requiring database backing, useful for validations and business logic. For example, you might use Active Model to represent a form object that doesn’t require a database table but still needs validations.
Active Record in Practice
Active Record tightly couples Ruby classes to the database schema. Each class represents a database table, and each object instance represents a row in that table. This means that attributes of an Active Record object map directly to the columns in the database, providing a way to manipulate data without writing raw SQL queries.
For example, imagine you have a Book
class that represents a table of books in your database. Each instance of Book
represents a single row, and the attributes (such as title
, author
, or published_year
) directly correspond to columns in the database. With Active Record, you can easily perform operations like:
- Creating a new record:
book = Book.create(title: "The Great Gatsby", author: "F. Scott Fitzgerald")
- Updating a record:
book.update(published_year: 1925)
- Deleting a record:
book.destroy()
Relationships between models can also be handled easily. For example, a Book
might belong to an Author
:
class Book < ApplicationRecord belongs_to :authorend
class Author < ApplicationRecord has_many :booksend
In this example, the Book
model includes a belongs_to
relationship with Author
, while the Author
model has a has_many
relationship with Book
.
All of these interactions happen without the need for direct SQL, as Active Record encapsulates the data access logic within these methods.
Active Record as an ORM Framework
As an ORM framework, Active Record offers the following capabilities to developers:
- Model Representation: classes represent models and their data.
- Association Representation: defines associations between models, such as
belongs_to
,has_many
, orhas_one
. - Inheritance Handling: thrtough inheritance, related models can share attributes or behavior.
- Validations: validates models before they get persisted to the database.
- Object-Oriented Database Operations: makes the code more readable and easier to maintain.