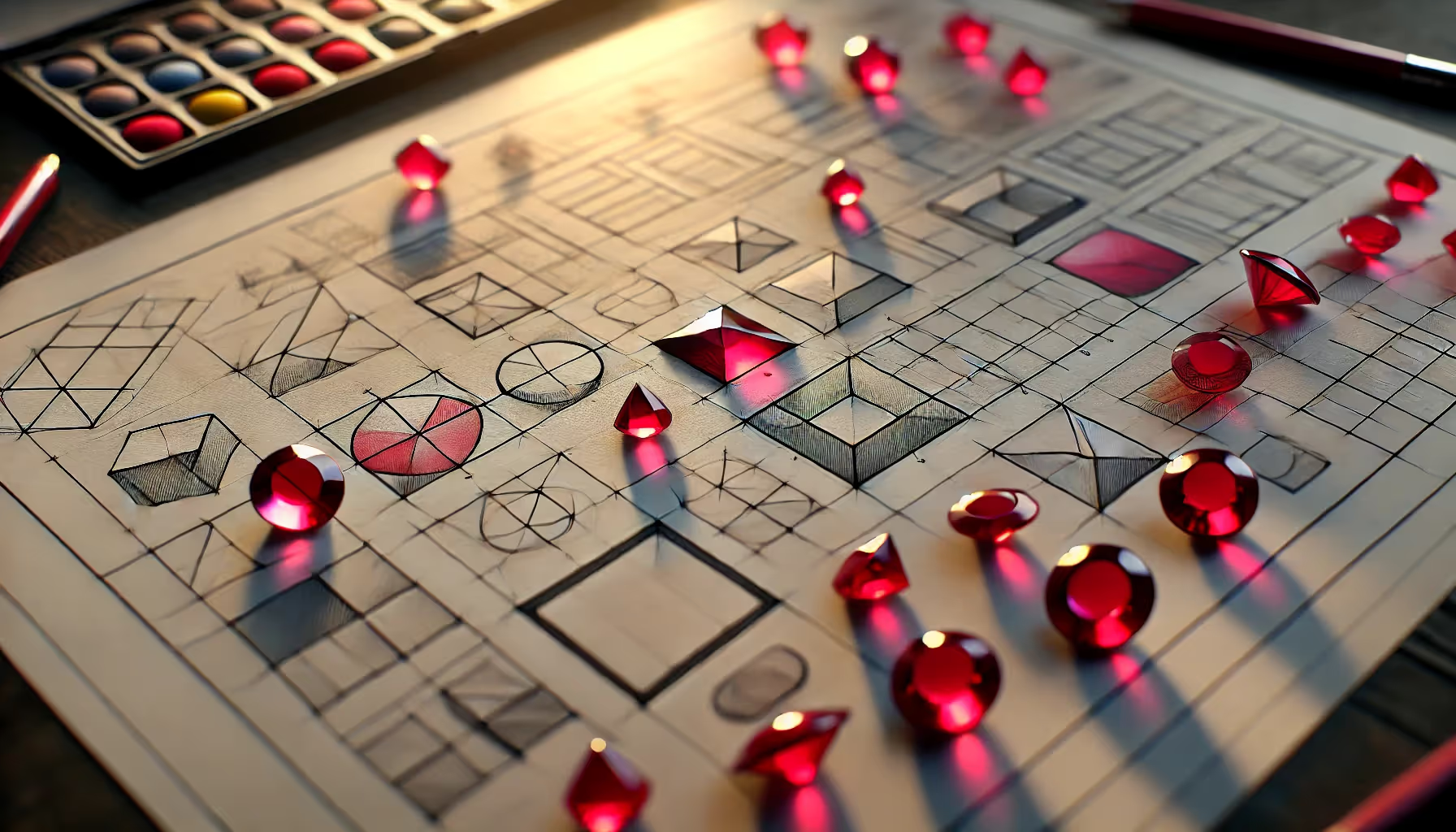
HTML is the essential piece to creating content for the web, but it’s a static markup language without backend logic. Historically, developers have embedded backend logic into HTML templates using technologies like Microsoft’s ASP or PHP. Ruby offers a similar solution called ERB, short for “Embedded Ruby.”
How to Use ERB Templates for Dynamic HTML
ERB allows Ruby code to be embedded directly within HTML templates. Here’s an example that list tasks for a project:
<html> <body> <h1>Tasks for project: <%= project_name %></h1> <ul> <% tasks.each do |task| %> <li><input type="checkbox"> <%= task %></li> <% end %> </ul> </body></html>
Here’s how it works:
- Ruby code within
<% ... %>
is executed without direct output. - Ruby code within
<%= ... %>
tags is executed and its output is embedded directly into the HTML.
So, if we remove the HTML markup and leave only the ERB content, it would be equivalent to the following:
puts project_name
tasks.each do |task| puts taskend
Rendering ERB Templates in Ruby: Step-by-Step Example
Let’s look at a complete Ruby script that shows how ERB templates are rendered:
require 'erb'
template = %( <html> <body> <h1>Tasks for project: <%= project_name %></h1> <ul> <% tasks.each do |task| %> <li><input type="checkbox"> <%= task %></li> <% end %> </ul> </body> </html>)
project_name = 'Fitness tracking app'tasks = ['Check pending pull requests', 'Deploy new features to staging', 'Update project documentation']
html = ERB.new(template).result(binding)
puts html
- First we require the
erb
library from the Ruby Standard Library. - Next, we define a template, which is just a variable containing a large string. Instead of using quotes, we are using the
%()
notation, so the quotes that the string may contain do not cause any conflict. - Then we define the two variables required by the template: a string, and an array of strings.
- And next we instantiate a new ERB object passing the template, and then calling the
result
method with abinding
parameter. The rendered output is stored in thehtml
variable. - The
binding
object is a method of the Kernel module. It provides the execution context in which the ERB template is evaluated. This means it makes local variables (and methods) defined in that scope available to the template. In our example,project_name
andtasks
are local variables that the template references. - Finally, the
html
variable contents are displayed.
This script outputs:
<html> <body> <h1>Tasks for project: Fitness tracking app</h1> <ul> <li><input type="checkbox"> Check pending pull requests</li> <li><input type="checkbox"> Deploy new features to staging</li> <li><input type="checkbox"> Update project documentation</li> </ul> </body></html>
Note that the Ruby code was processed and the variables were used.
Test your knowledge
-
What does ERB stand for in Ruby?
-
Which ERB tag executes Ruby code without directly outputting anything to HTML?
-
What does the
<%= ... %>
ERB tag do?
-
What is the purpose of the
binding
parameter in ERB?
-
What will the Ruby method
ERB.new(template).result(binding)
return?